Published on: 10/7/20 2:52 AM
Category:Express Node Tags: Express, Node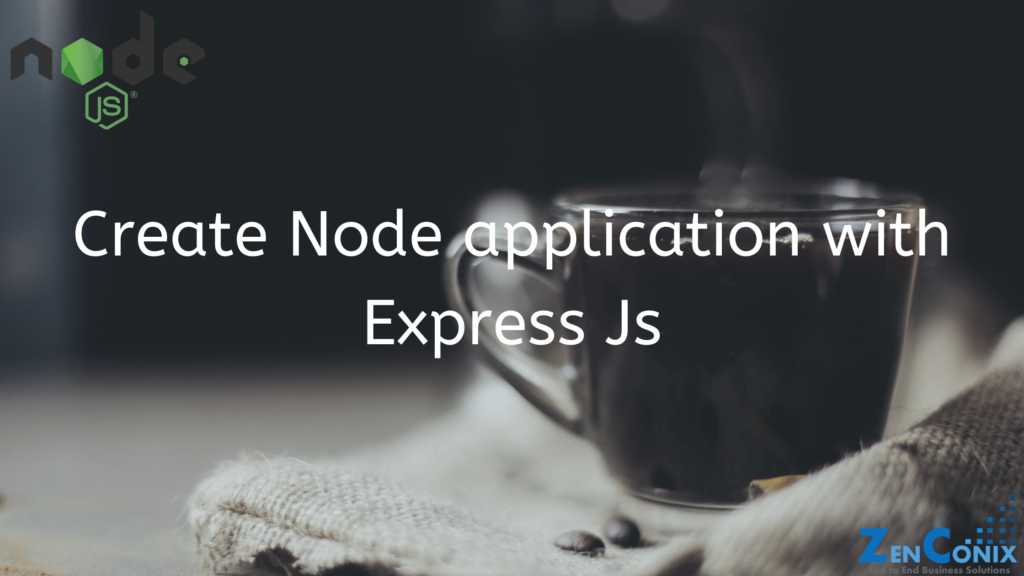
In this post, we are going to take a look on how to create Node application with Express JS.
You need to install node and npm in your machine.
Node can be installed from https://nodejs.org/en/download/
npm can be installed from https://www.npmjs.com/get-npm
To create application, open terminal and enter command npm init
It will ask you series of questions, you just have to answer them or can just press enter button to take its default value.
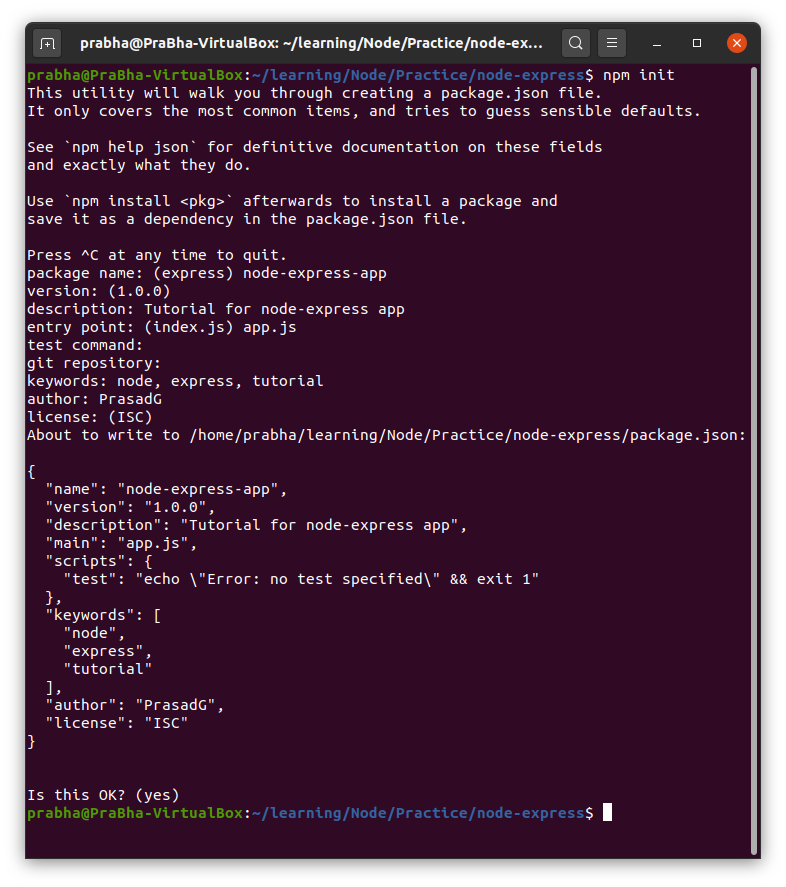
here, I just added entry point as app.js
, you can keep it anything you want or even index.js
also should be fine.
Once you enter Yes, it will create new file in selected folder named package.json
, this file contains everything which we have mentioned above, packages information which we required to installed in app and some other configuration which we will required for future .
{
"name": "node-express-app",
"version": "1.0.0",
"description": "Tutorial for node-express app",
"main": "app.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [
"node",
"express",
"tutorial"
],
"author": "PrasadG",
"license": "ISC"
}
Next step, we need to install Express JS package by npm.
To install, express package open terminal and enter command
npm install express --save
this will install express package to our application and so it will update the package.json
file as well.
New dependency is added in package.json
file.
"dependencies": {
"express": "^4.17.1"
}
Also it has created new folder named “node_module” which contains express js plugin and its dependent plugins.
Now entry point for application, as we have mentioned our entry point will be app.js, we will create a file named app.js.
First step in app.js file is, we need to include module for express. To do it, simply write as below.
const express = require('express');
Second we need to initilize the express module, to do this, add below line of code
const app = express();
Then we need to listen the port
Here we need to specify the port number, it can be anything as you want. I have added as 3000.
app.listen(3000);
Finally, our complete app.js file will look as below.
const express = require('express');
const app = express();
console.log("app is running");
app.listen(3000);
Here I just added console to check the output in console.
To run this app, open terminal and enter run the application by
node app.js
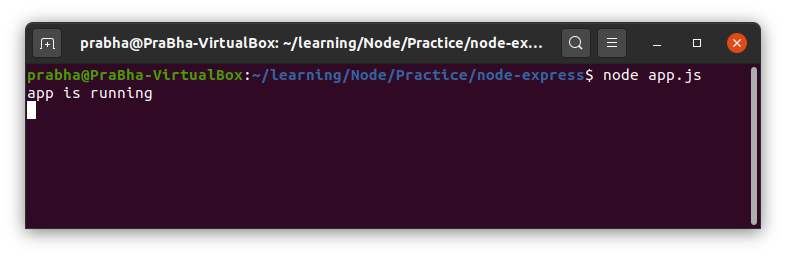
Our express app is running on port 3000.
By this way, we can create node application with express js.
In upcoming posts, we will take a look deeper into express js and how to work with it.
Happy Coding !