Published on: 10/7/20 6:10 AM
Category:Express Node Tags: Express, Node, routers, routes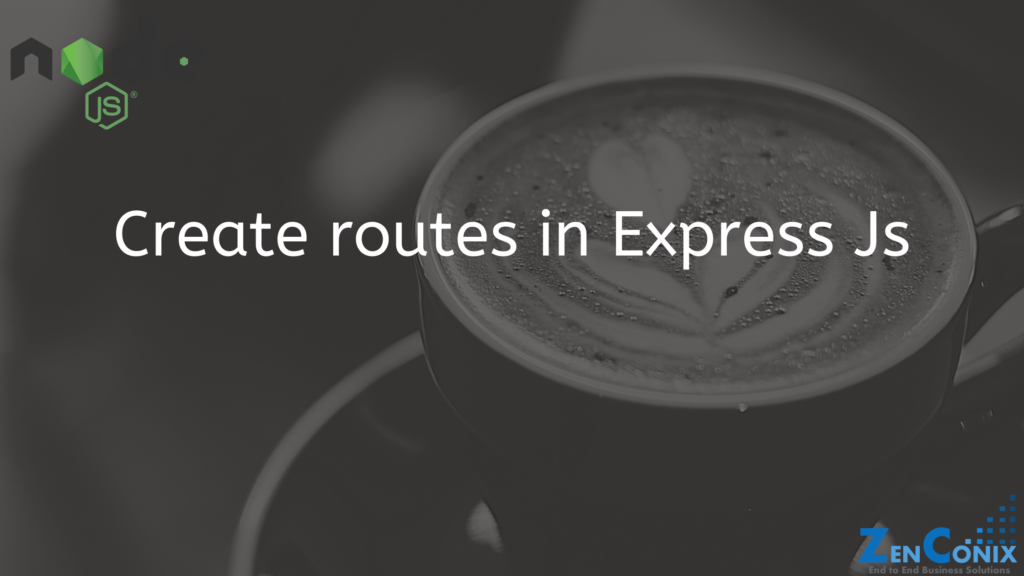
In last post, we have seen how to create express js application in node.
http://zenconix.com/create-node-app-with-express-js/
In this post, we are going to take a look on how to create routes in express application.
To add routes in our application, we need to add middlewares, detailed information about middlewares in express js is available at the link
https://expressjs.com/en/guide/writing-middleware.html
to add routes in our file, we can use “use” middleware or also can use http method which middleware applies. e.g. get/ post etc.
Following figure shows elements of middleware function calls;
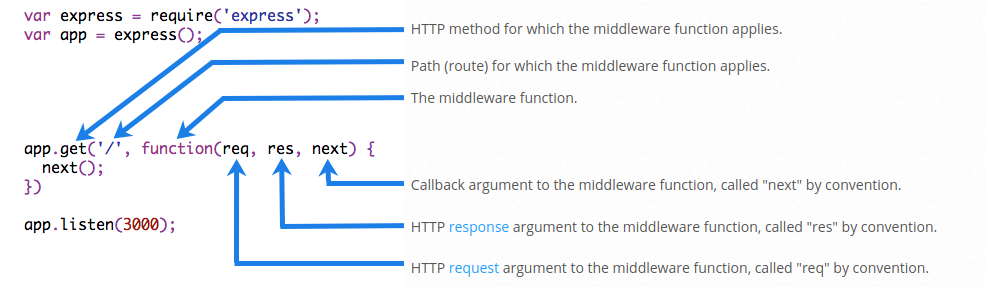
https://expressjs.com/en/guide/writing-middleware.html
lets take an example of using “use” middleware
app.use('/', (req, res, next) => {
res.send('<h1>node application is running</h1>');
})
run the node application using node app.js
command
Open the browser, and enter url as http://localhost:3000/
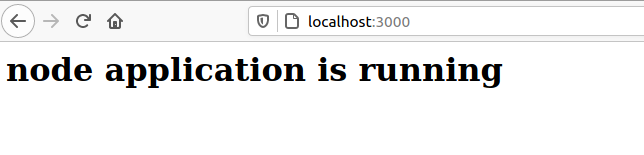
Also if we check the headers for this request, you can see it has automatically taken method as GET method.
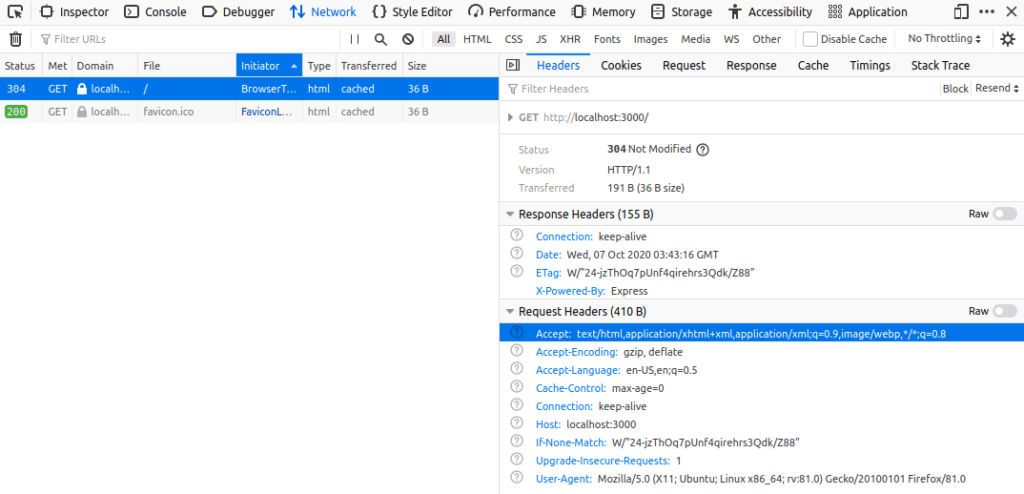
Instead of using “use” middleware, we can also use http method specific middleware to add routes in application.
Let’s take a look at GET method
app.get('/data', (req, res, next) => {
res.send('<h3> This is GET route </h3>');
})
Now stop the application and run it again using node app.js
command
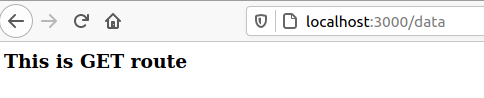
Now, check the header response , there we can see method is taken as GET and content-type
is set to text/html
automatically
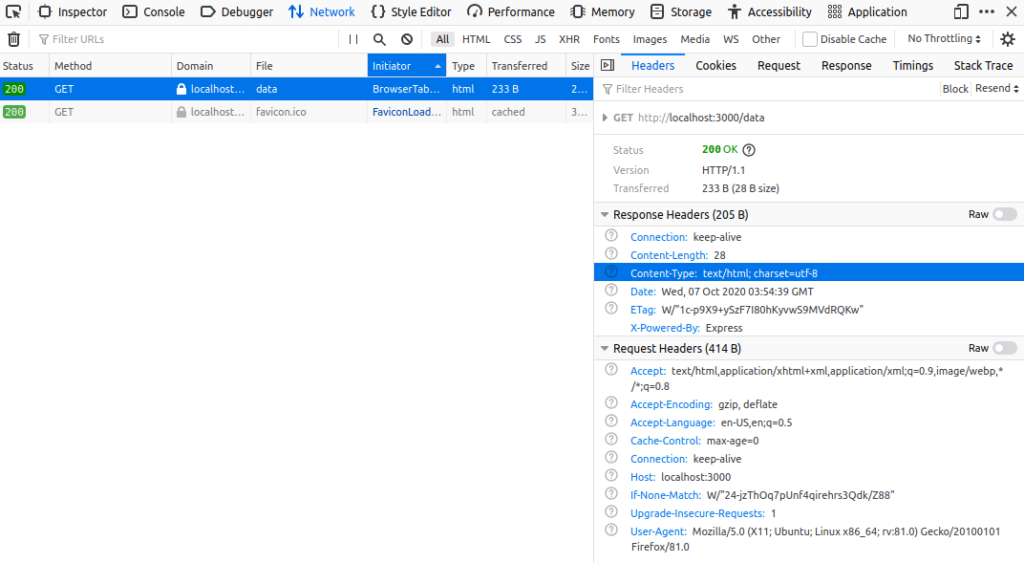
Similarly POST request can be added as below.
To create a post request, we will first create form which will be used to add some data.
app.get('/add-data', (req, res, next) => {
res.send(
'<form action="/data" method="post"><input type="text" name="name"> <button type="submit">Submit</button></form>'
);
});
Above is get router, to display the form and we have added action="/data"
for form, so once it is submitted, it will redirect the page to /data
route.
Now we will add Post route to get the data
app.post('/data', (req, res, next) => {
console.log(req.body);
})
Now lets try to run the application by node app.js
and go to url
http://localhost:3000/add-data
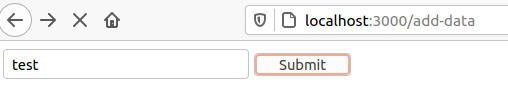
Enter some data and submit and check for our console.
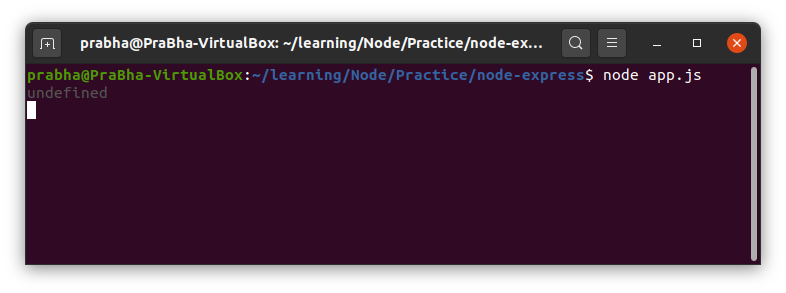
It has shown the data as undefined, this is because express app is not parsing the request body, to do that we need to parse the body.
We have plugin for body parsing, we will install that plugin.
npm install body-parser --save
now we need to add module to our app.js file.
const bodyParser = require('body-parser');
app.use(bodyParser.urlencoded());
now run the app by entering command node app.js
in terminal and go to http://localhost:3000/add-data
add some data textbox and hit enter and check console.
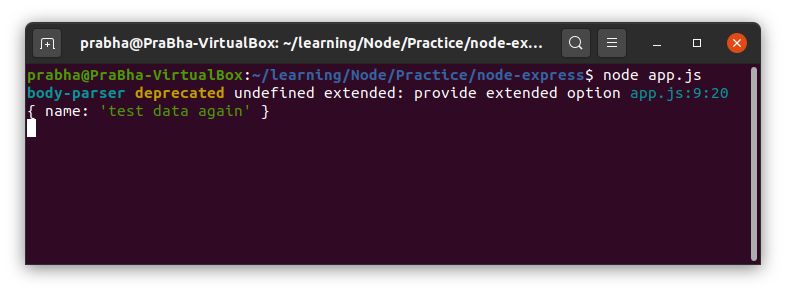
As we are getting this error in console, add extended property to body parser.
app.use(bodyParser.urlencoded({extended: true}));
and run again using node app.js
command in terminal, go to http://localhost:3000/add-data
add some data textbox and hit enter and check console.
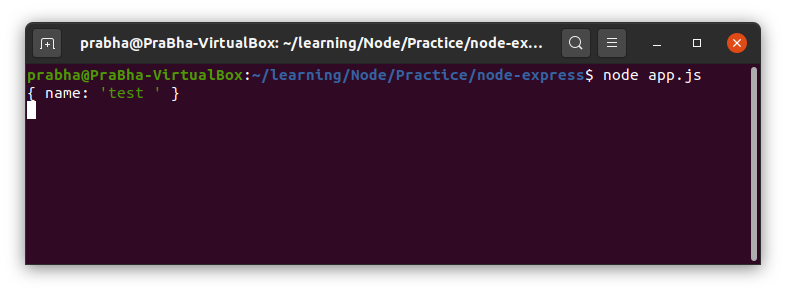
As we have seen we can write our routes in app.js file but by doing this, our app.js file will be more bigger and not readable, so it is best practice to separate out this routes to elsewhere.
So lets create a folder name “Routers” in root folder of our application.
In “routers” folder, create two js files named admin.js
and user.js
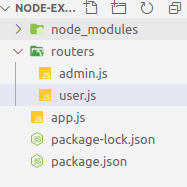
admin.js
file will contain all routes required for admin role and user.js
file will contain all routes required for user role.
Let’s create routes for user first
Go to user.js
file, add express module and then initialize Router function from provided by express js as below.
const express = require('express');
const router = express.Router();
move our get route to user.js
file as below
router.get('/data', (req, res, next) => {
res.send('<h3> This is GET route from user js </h3>');
});
Note: instead of using app.get
, here we have use router.get
function because we have initialize the router middleware here.
Finally, we need to export the router so that we can use that in another file using require function.
module.exports = router;
Our complete user.js
file will look as below.
const express = require('express');
const router = express.Router();
router.get('/data', (req, res, next) => {
res.send('<h3> This is GET route from user js </h3>');
});
module.exports = router;
To use user.js file’s route, we need to import this file to app.js
First import the module, as it is not core module, we need to give full path for module as below.
const userRoutes = require('./routers/user');
Then we need to pass this to middleware as below
app.use(userRoutes);
Again run our node app by entering node app.js
command in terminal and go to http://localhost:3000/data
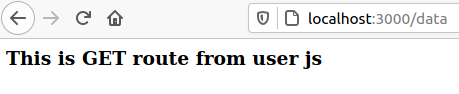
Similarly, we can move our admin routes to admin.js file, our admin js
file will be as below
const express = require('express');
const router = express.Router();
router.get('/add-data', (req, res, next) => {
res.send(
'<form action="/data" method="post"><input type="text" name="name"> <button type="submit">Submit</button></form>'
);
});
router.post('/data', (req, res, next) => {
console.log(req.body);
})
module.exports = router;
And accordingly, we need to import admin routes in our app.js
file
const adminRoutes = require('./routers/admin');
app.use(adminRoutes);
Now, run our node js application again by entering command in terminal as node app.js
and go to browser and enter urls you will get same result as before.
Finally we will see if there is unwanted router comes , then we need to throw a page for 404 not found.
Go to app.js file and add use middleware
app.use((req, res, next) => {
res.status(404).send('<h3> 404 page not found, please try with different URL </h3>');
})
Now go to browser and add any URL which we have not yet handled in router.
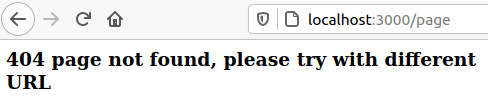
also you can see status is shown as 404 in network tab
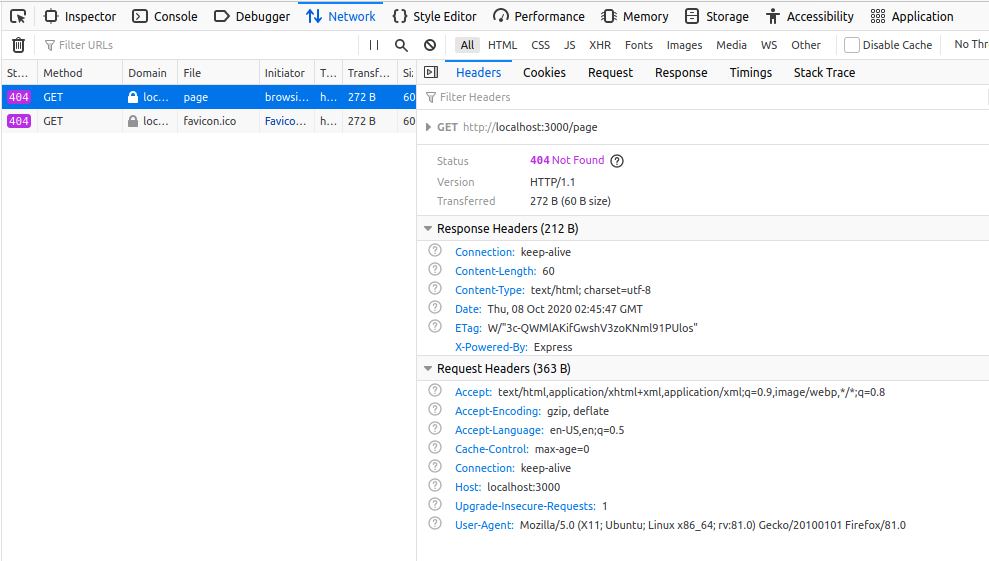
I hope this explained you , how to add routes in Express js.
Happy Coding 🙂
Saw your material, and hope you publish more soon. Nada Benjamen Pavkovic